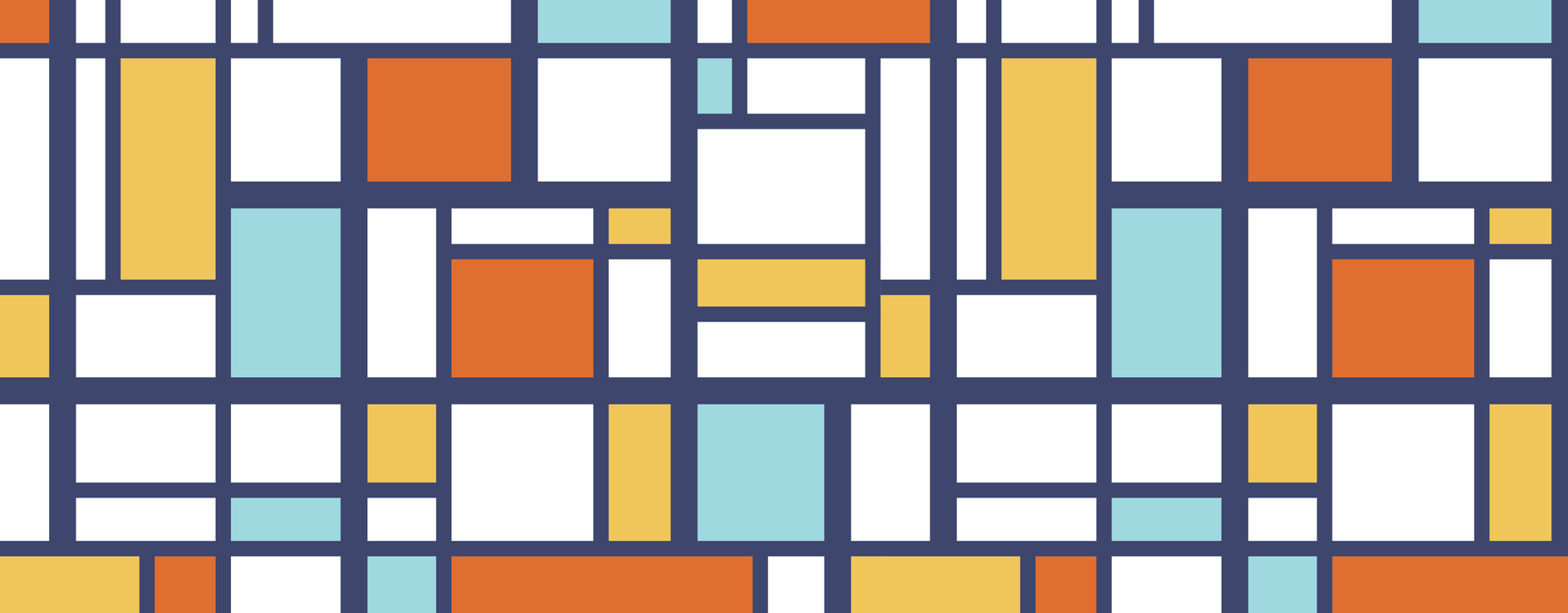
Getting started with CSS Grids
The CSS Grid Layout is the first CSS module designed specifically to create layouts. It provides a set of properties, functions and flexible units that allows you to control the sizing, positioning and spacing of content.
Throughout history we, the front-end developers, have been hacking our way around the limitations of CSS layouts to create grid based designs. First we used tables, then floats, positioning and inline-blocks. Then Flexbox came, but being a one-dimensional layout tool, meaning it aligns content and distributes space along one single axis (a row or a column), it didn’t worked quite right when building more complex two-dimensional designs. In the other hand the CSS Grid Layout (or just “Grid”) lets us skip all these workarounds, by providing new properties that allows us to control the aspects of rows and columns at the same time.
Defining Grids
To get started you have to define your **grid container**, which establishes the grid formatting context and manages how its child elements are sized, spaced and aligned. When defining the grid container automatically all its direct children become **grid items**.
For example, here’s the markup for a grid container containing six grid items:
<div class="grid">
<div class="child">1</div>
<div class="child">2</div>
<div class="child">3</div>
<div class="child">4</div>
<div class="child">5</div>
<div class="child">6</div>
</div>
To turn our grid container into a grid, we just give it the value of `grid` on the display property:
.grid {
display: grid;
}
¡Congrats! You’ve created your first grid. This is what it looks like:
See the Pen NBmVPz by Tomas Peralta (@tomasperalta) on CodePen.
You probably noticed that the result doesn’t look much like a grid. That’s because, by default, the grid container places all its grid items in a single column. In order to place the grid items into multiple columns we need to set the value of the `grid-template-columns` property. For example if we would like to have a three-column layout, we need to set three values for the `grid-template-columns` property. I’ll start setting each column size to 250px pixels wide.
.grid {
display: grid;
grid-template-columns: 250px 250px 250px;
}
This is what your grid should like:
See the Pen GXJoJv by Tomas Peralta (@tomasperalta) on CodePen.
So, what about rows? We haven’t explicitly defined the rows of our grid yet. By default rows are as tall as the content they hold, but we can control how much vertical space they take by setting values to the `grid-template-rows` property. For example, to set the first row to 50 pixels and the second one to 100 pixels tall we should do this:
.grid {
display: grid;
grid-template-columns: 250px 250px 250px;
grid-template-rows: 50px 100px;
}
With CSS Grids we have total control over the spaces, or gutters, between columns and rows. We separate grid tracks using the `grid-column-gap` and `grid-row-gap` properties (or just the `grid-gap` property if the two gaps are the same). Let’s add a 20 pixel gutter to our grid:
.grid {
display: grid;
grid-template-columns: 250px 250px 250px;
grid-template-rows: 50px 100px;
grid-gap: 20px;
}
And this is the result:
See the Pen GXJoQx by Tomas Peralta (@tomasperalta) on CodePen.
Congratulations! You’ve just setup your first CSS Grid layout. Easy peasy, right?
In the past we would have to rely on other methods like positioning, inline-blocks and flexbox to achieve this grid layout. Now we only have to use a few hand of the properties that the CSS Grid Layout provides.
#### Where is my flexibility?
You probably noted that the grid layout we defined earlier has fixed values for its columns and rows, which isn’t of much help on terms of responsiveness. Helpfully CSS Grids introduces new features that intelligently adapts and positions the content to available space.
Grids provides a flexible unit designed to create grid tracks that expand or contracts based on the available free space in the grid. For example if you see our grid layout, there is free space after the last column. This is because the sum of the columns’s width isn’t enough to cover the whole grid width. Let’s make our grid flexible using de `fr` unit, where “fr” stands for _“fraction of the available space”_. For example if we set the second column width to one fr, it will expand to fill up that extra space.
.grid {
display: grid;
grid-template-columns: 250px 1fr 250px;
grid-template-rows: 50px 100px;
grid-gap: 20px;
}
Now the middle column is flexible, while the first and last column are fixed at 250 pixels.
As you can see the `fr` unit removes the need for math. Before CSS Grids, we would have to use the CSS `calc()` function to calculate the available free space, including the fixed values and even the gutter space, and a little flexbox to distribute the content along the row.
Let’s quickly set our columns to take exactly 1 fraction of the available space:
.grid {
display: grid;
grid-template-columns: 1fr 1fr 1fr;
grid-template-rows: 50px 100px;
grid-gap: 20px;
}
Now we have a flexible, equal-width, three column layout.
Another handy feature that CSS Grids provides is the `repeat()` function, which helps you to save time and keep your CSS maintainable. This function serves as a shortcut for repeating patterns of tracks, which keeps you from having to write the same values over and over again. It can be used in the `grid-template-columns` as well in the `grid-template-rows` properties.
For example we have a layout that consists in three equal-width columns, that we’ve set up using three `1fr` values. This could be simplified by the `repeat()` function, just pass it the repetition value (in this case 3) followed by a comma and the value to repeat (in this case `1fr`):
.grid {
display: grid;
grid-template-columns: repeat(3, 1fr);
grid-template-rows: 50px 100px;
grid-gap: 20px;
}
And that’s the basics on creating grids using the CSS Grid Layout!
We didn’t covered all the concepts that Grids provides, but it should be enough for you to start creating layouts today! Also, here is a helpful cheatsheet with visual examples of the CSS Layout properties for you to check out.